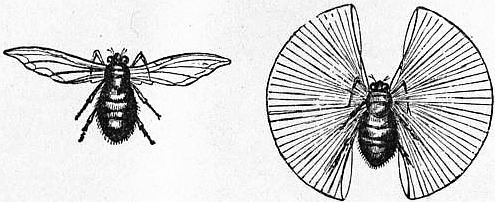
Easy Patterns in Python: The Flyweight Pattern
Introduction The flyweight pattern is a pattern that helps minimize memory usage by sharing and reusing data. A...
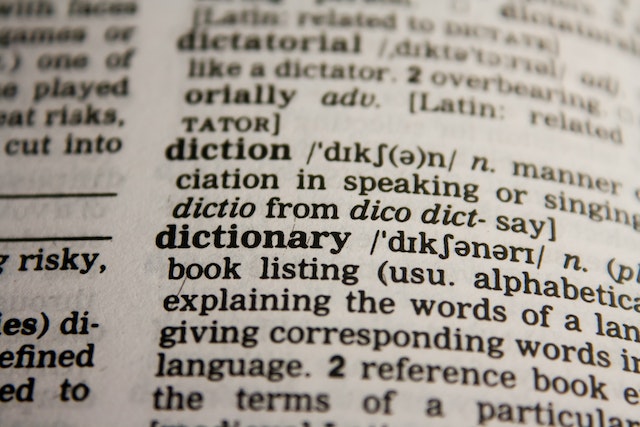
Easy Patterns in Python: The Interpreter
Introduction The Interpreter pattern can be used to interpret and evaluate sentences in a language. The idea is...
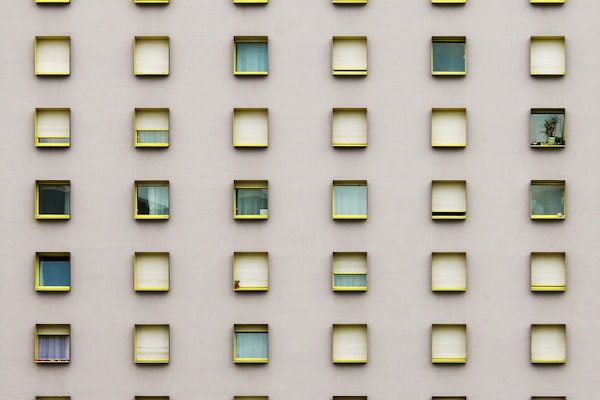
Easy Patterns in Python: the Facade
Introduction The facade pattern is used as a way to hide more complex logic. A facade can do...
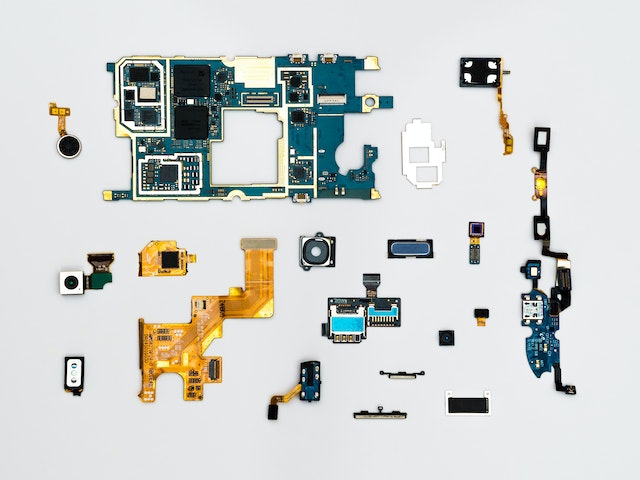
Easy Types and Generics in Python: the Prototype Pattern
Introduction The prototype-pattern is a creational design pattern that allows us to create new objects by cloning existing...
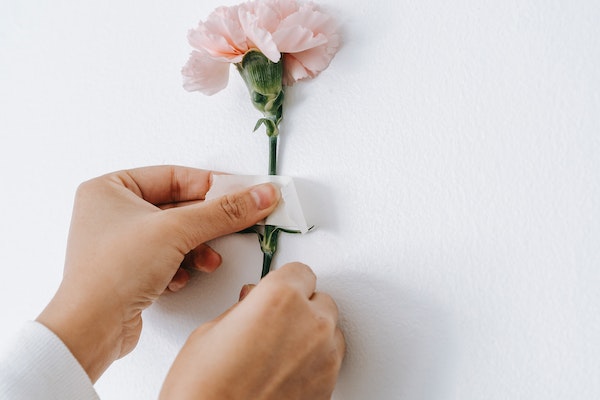
Easy Python Patterns: The decorator pattern
Introduction The Decorator pattern can be used to dynamically alter or add functionality to existing classes. This pattern...
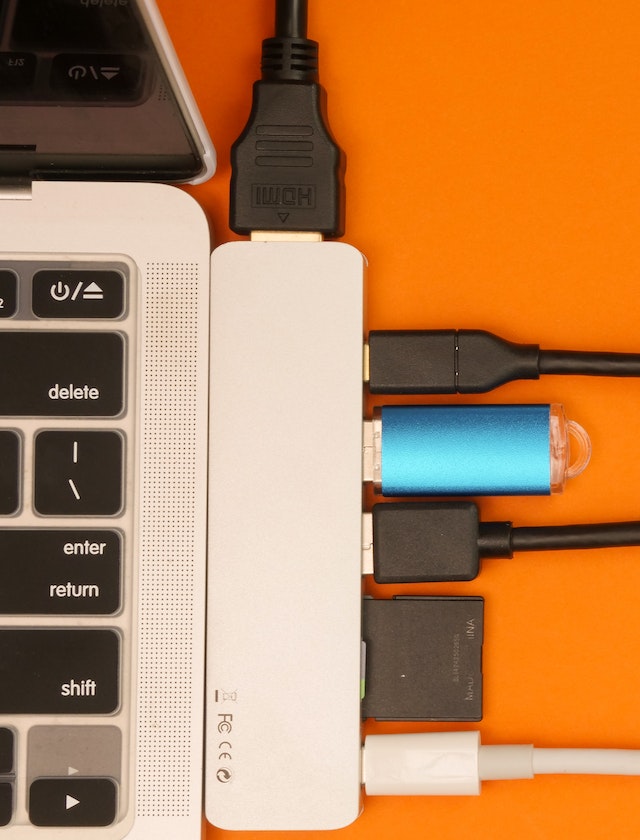
Python patterns for fun and profit: The Adapter Pattern
Introduction The Adapter pattern is used to make one interface compatible with another. It allows objects with different,...
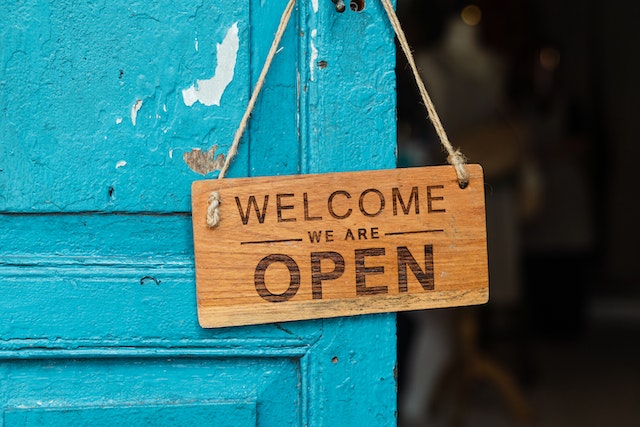
Using Python types for fun and profit: the Visitor Pattern
Introduction The visitor pattern is a design pattern that allows for adding new operations to a collection of...
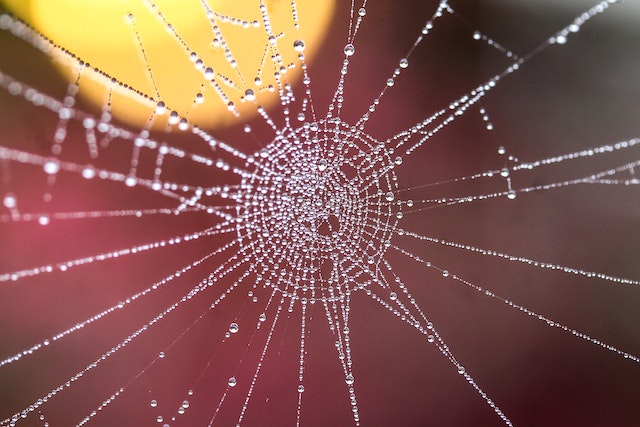
Using Python types for fun and profit: the Mediator pattern
Introduction The mediator pattern is a pattern used when you want to simplify communication i.e. message dispatching in...
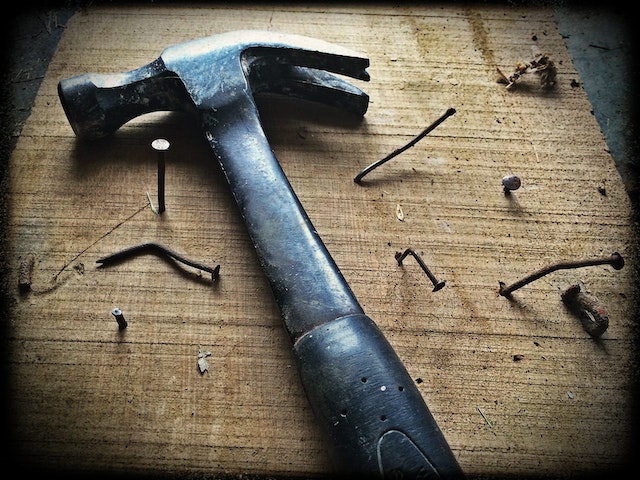
Using Python types for fun and profit: the Builder
Introduction The builder pattern is a creational design pattern, i.e. it is a pattern for creating or instantiang...
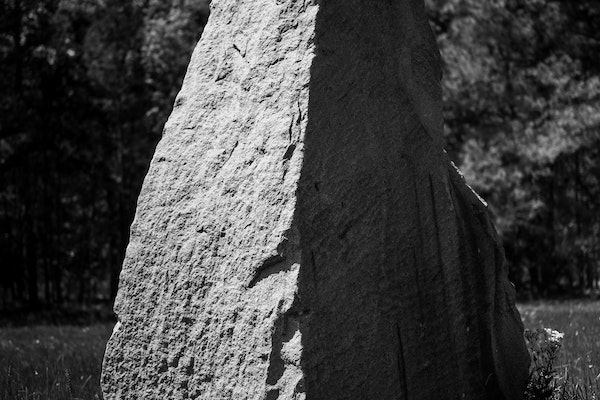
Using Python types for fun and profit: the Singleton
Introduction The singleton pattern restricts the instantiation of a class to a single instance. The singleton pattern makes...