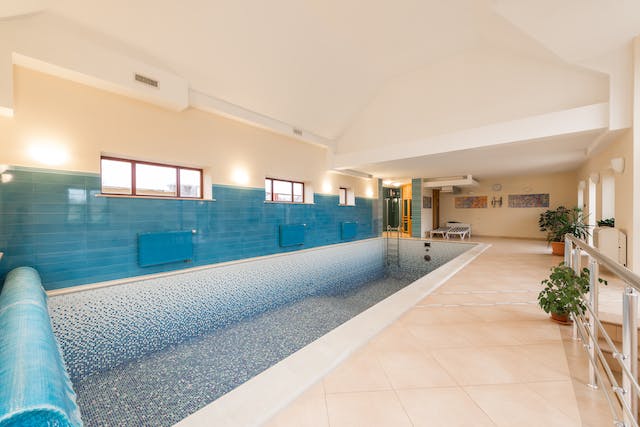
How to Create an Easy Thread-Safe Object Pool in Python with ContextManager
Introduction Sometimes, to save time and resources, it’s handy to have a collection of ready-to-use items, which we...
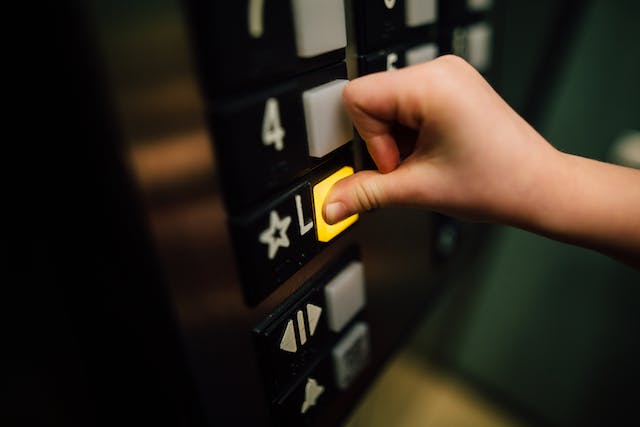
Mastering the Easy Art of Delegation in Python: A Step-by-Step Guide
Introduction Delegation is like passing a job to someone else. In Python, you can do this by using...
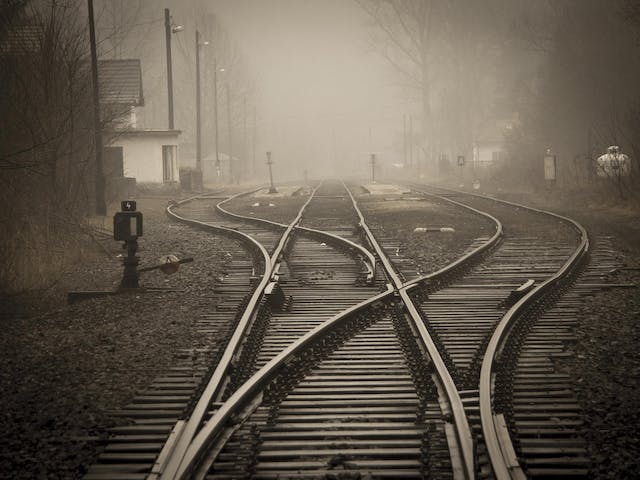
Making Resource Acquisition Easy: Python’s Simple Guide to RAII
Introduction Resource Acquisition and Initialization means the following: There are several ways to implement this pattern in Python:...
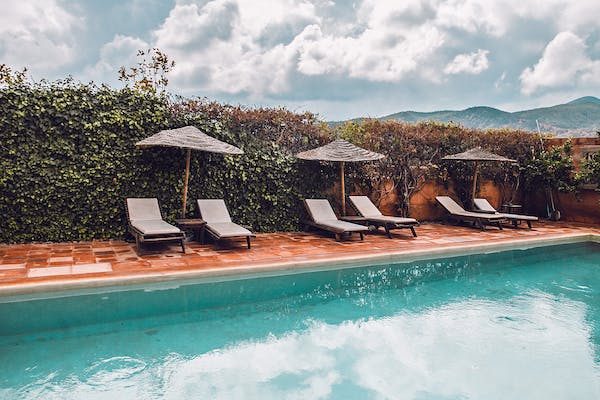
Simple Resource Management: Implementing the Object Pool Pattern in Python
Introduction Sometimes, for the sake of efficiency, it can be really helpful to keep a group of ready-to-use...
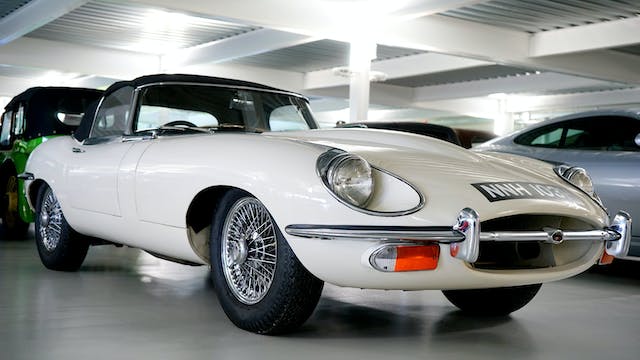
Easy Implementation of Python’s Lazy Initialization Pattern for Improved Efficiency
Introduction Sometimes, making something can be costly. It might take a lot of resources or time to create...
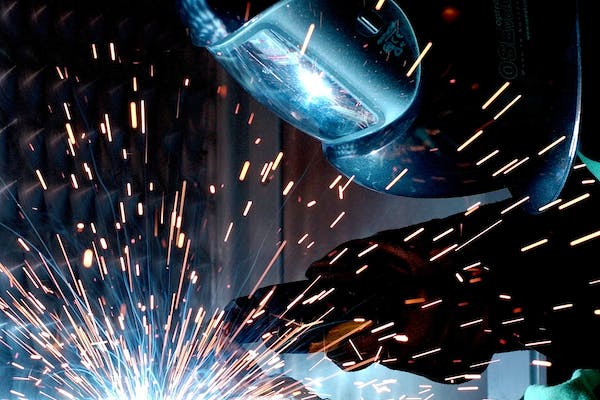
Unlocking the Magic: Mastering the Factory Method in Python
Introduction In this article, we’ll explore a concept called the Factory Method in Python. The Factory Method is...
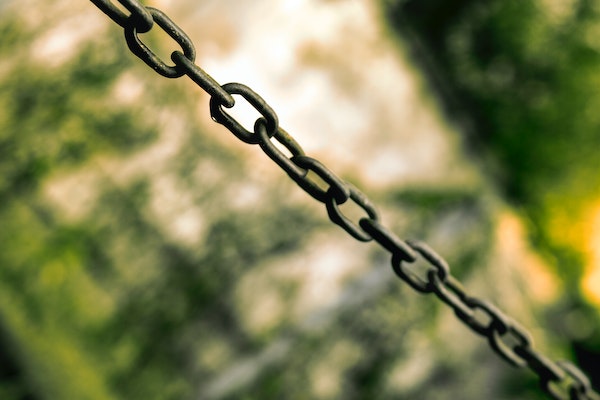
Easy patterns in Python: Chain of Responsibility
Introduction The Chain of Responsibility pattern describes a chain of command/request receivers. The client has no idea which...
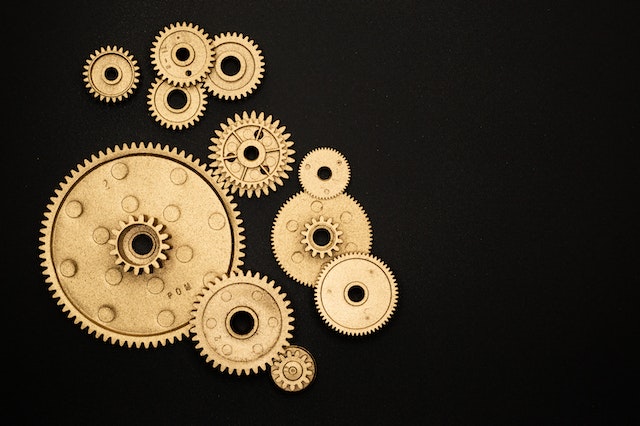
Easy patterns in Python: The Composite Pattern
Introduction The composite pattern allows you treat a group of objects like a single object. The objects are...
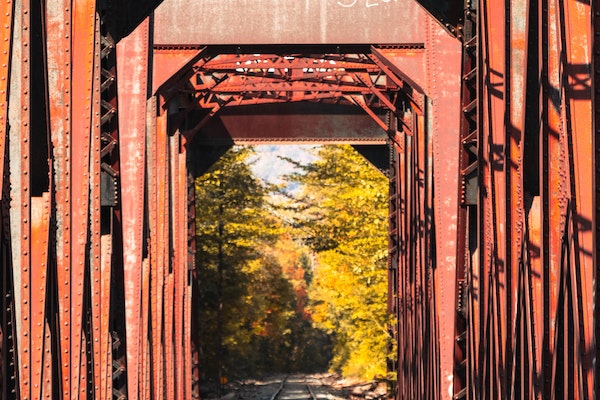
Easy Patterns in Python: The Bridge
Introduction The Bridge pattern is a design pattern that is meant to “decouple an abstraction from its implementation...
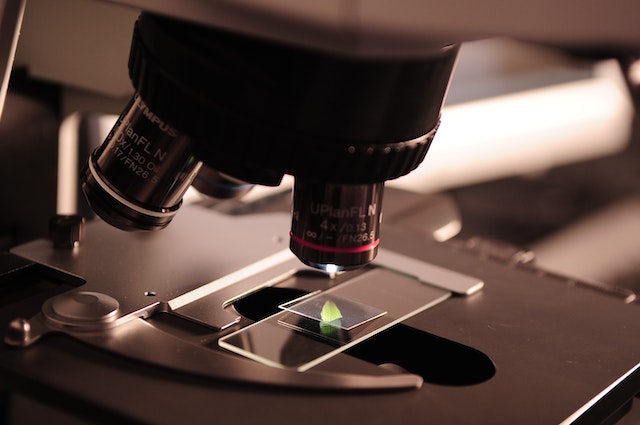
Easy Python Patterns: The Observer pattern
Introduction The observer pattern is a software design pattern that allows an object, usually called the subject, to...